Courses
CSS 51 Computing Laboratory (click for details)
List of Experiments
Assignments on expression evaluation
Assignment-1
Write the pseudocode and draw a flowchart:
- To find the sum of 5 numbers
- To compare between two numbers and print the maximum number.
- To print “Hello good morning”.
- To take the marks of 5 subjects of a student. Compute the total and Average. If the average is less than 40, display FAIL', otherwise display 'PASS". Then check if the average is less than 50, display D' otherwise, check again. If the average is less than 60, then display C' otherwise, check again. If the average is less than 70, display B otherwise, check again. If the average is less than 80, display 'A' otherwise, display ‘EX’.
Implementation of conditional branching (if, if-else, switch case)
Assignment-2
- WAP in C to swap two numbers with/without using a third variable.
- Write a C- program to generate the following series using if else. 0 1 1 2 3 5 8 13
- WAP to print a number is Even or Odd using if else.
- WAP to find out biggest among 3 numbers taken from keyboard using if else.
- WAP a program to take a character from keyboard and check whether it is vowel or not. If the character taken from keyboard is small, then display its corresponding capital value and vice versa.
Implementation of iterations (for, do while, while)
Assignment-3
- WAP to find out the max number in an array of 10 integers.
- WAP to take an array of 5 integers from keyboard and print the sorted array i descending order.
- WAP to take an array of 10 floats and print reverse of the array.
- WAP to take the marks 5 subjects of a student in an array. Compute the total. If the total is more than 40 and display the student has Passed otherwise display fail.
- WAP to take enter 20 numbers from keyboard to an array. The number to be searched is also entered from the keyboard. Find out if the entered number is present in the array or not. If it is present, display the number of times it appears in the aray.
- WAP to enter values in two separate 6X6 matrix find add and difference (minus) of two 6X6 matrices.
- WAP to enter values in two separate 4X4 matrix and find multiplication of the two 4X4 matrices.
- WAP to create a multiplication table using a 2-D array and display the same.
- WAP to take marks of 10 students in an array. For each student individual marks for 5 subjects need to be entered in an array. Calculate the sum and average marks for each student and display.
- WAP to find if a square matrix is symmetric or not.
Implementation of function and recursive function
Assignment-4
- WAP in C to find out factorial of a number.
- Write a C- program to generate the 20 numbers in a Fibonacci series 0 1 1 2 3 5 8 13
- WAP in C to print reverse of a number. 4. WAP to print a number is Palindrome or not?
- WAP to print a number is Armstrong or not? 6. WAP in C to print sum of digits of a positive number.
Implementation of 1-d array and 2-d array
Assignment-5
- WAP to find out the factorial value of a number using function.
- WAP to pass two parameters in a function and return the maximum among them.
- Find out sum of numbers S =1+2+3+4+...+n using function.
- WAP to generate a menu like:
- Addition
- Subtraction
- Multiplication
- Division
- Even and Odd
- WAP to create a function that swaps between two integer values and shows the answer.
Implementation of pointers and parameter passing techniques
Assignment-6
- Write a program in C to find combination (𝑛𝐶𝑟) of a number using function.
- Write a program in C to calculate x to the power n (𝑥𝑛) using recursion.
- Write a program in C to calculate GCD and LCM of two numbers using recursion.
- Write a program in C to convert a decimal number to binary using recursion.
- Write a program in C to check a number is a prime number or not using recursion
- Write a program in C to check a number is a strong number or not using function.
- Write a program in C to multiply the elements of two matrices using functions.
Implementation of string handling using array and pointers
Assignment-7
- Write a program in C to develop your own functions xstrcat ( ).
- Write a program in C to develop your own functions xstrcmp().
Implementation of structure and union
Assignment-8
Structure and Union:
- Define a structure name “customer” which has the following fields.
- Account no -a positive integer.
- Name -a string of 25 character.
- Balance -a real values.
- Account type -a character.
- Write a C program to add two distances in feet and inches using structure.
- Write a C program to calculate total payment of workers.
- Write a program to generate data for N students. Use structure to create numeric ID and points (max 100) as 2 separate members. Randomly generate data for N students. Display both the ID and the points of the student who has received highest point.
- Write a C program to arrange student record in descending order.
- Define a structure type book that would contain book name, author, pages and price. Write a program to read this data using member operator (‘.’) and display the same.
Implementation of file handling
Assignment-9
- Write a program in C to read the file and store the lines in an array.
- Write a program in C to write multiple lines to a text file.
- Write a program in C to find the number of lines in a text file.
- Write a program in C to read an existing file.
- Write a program in C to write multiple lines to a text file.
LAB MANUAL
Central License/Open Source Software
Using SSL for secure web applications(Purchase from GlobalSign)
Using VPN for securely access internal resourse from outside of the campus(Purchase from SOPHOS)
Licenced Software
- ANSYS(v2022R2)
- MATLAB(vR2022b)
- Discovery Studio(v22.1.0.21297)
- COMSOL(v6.0)
- SPSS(v29.0.0.0)
Cloud based services
- Google G-suit gmail
- Microsoft Office 365
Open Source Software
- Apache (v2.4.35)
- Mysql for Database Server(v8.0.25)
- Tomcat(v8.0.2)
- Postgres sql for Database Server(v4.0.3)
- Squid service for Proxy Server(v4.9)
- Bind service for DNS(v8.3)
Facilities of Computer Center
Helpline
0343-275-2126
9434788004
For Network Issue email to
net.support@nitdgp.ac.in
For Central Software/Server Access Facility Issue email to
server.support@nitdgp.ac.in
For very emergency perpose contact/whatsapp to
9434788004
For Network Issue email to
net.support@nitdgp.ac.in
For Central Software/Server Access Facility Issue email to
server.support@nitdgp.ac.in
For very emergency perpose contact/whatsapp to
9434788004
Central Software Support
- ANSYS(v2022R2)
- MATLAB(vR2022b)
- Discovery Studio(v22.1.0.21297)
- COMSOL(v6.0)
- ABAQUS
- SPSS(v29.0.0.0)
- Cmake
- LAMMPS
- Gaussian 09
- CFOUR
- Intel Fortan/C Compliers with MKL Library
- MPI
- Gnuplot
Central System Information
Raise a Complaint
Staff Under Computer Center
Computer Center Staff
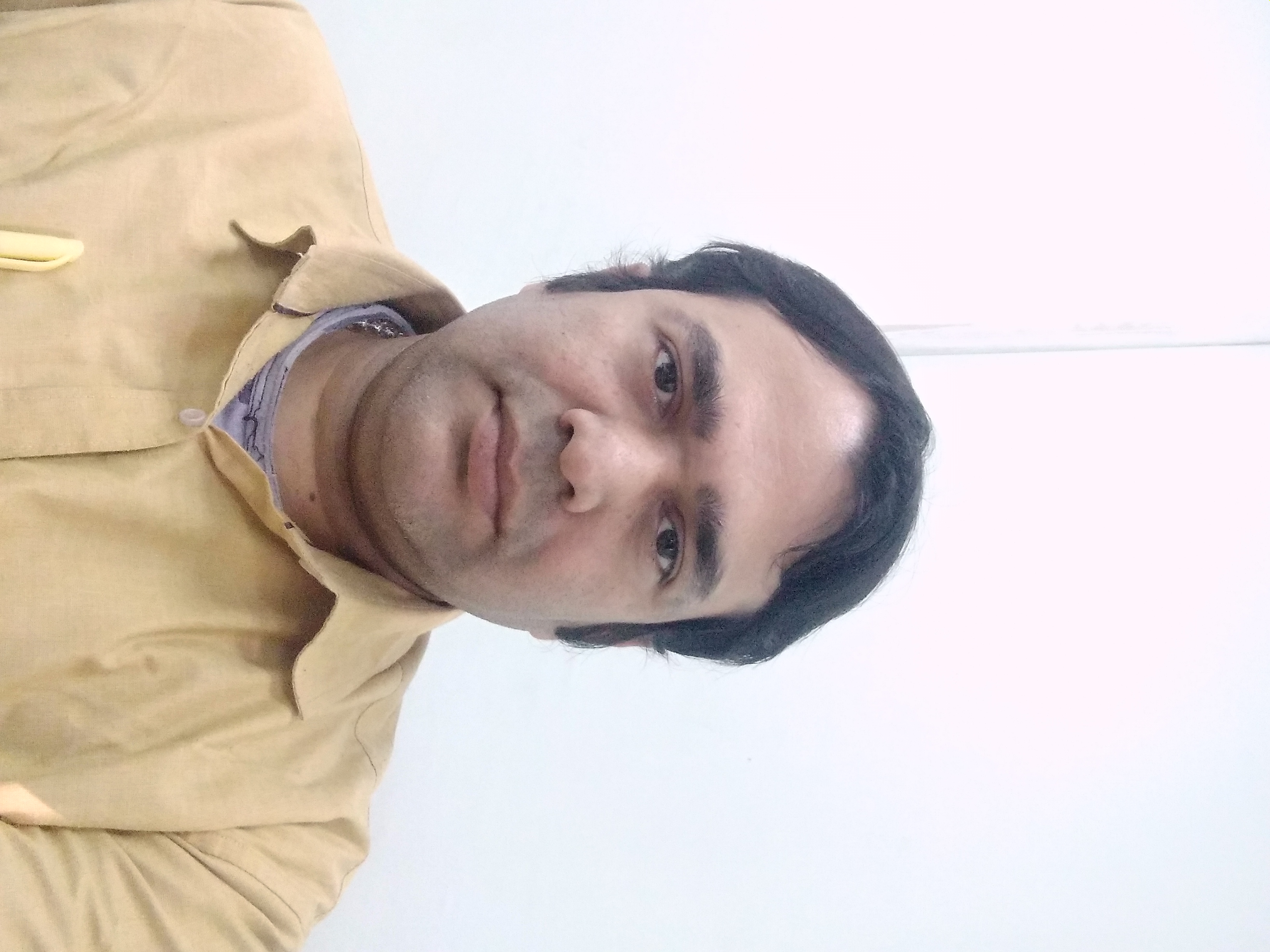
Avijit Kar
System Admin
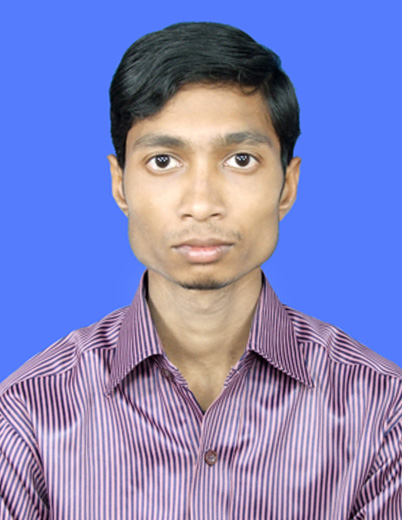
Rana Gorai
Technical Staff
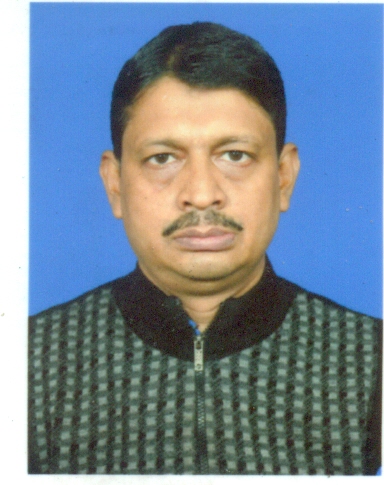
Debdash Sharma
Office Staff
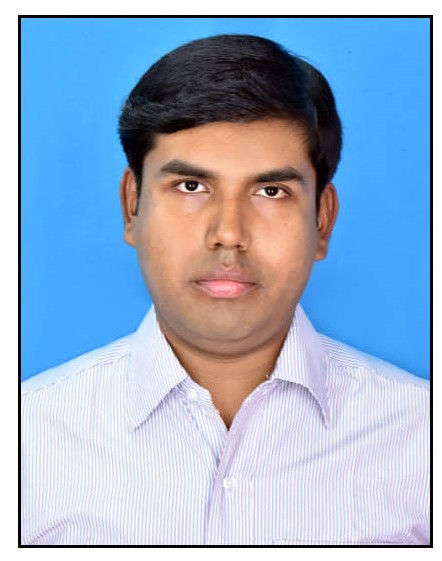
Somnath Saha
Office Staff
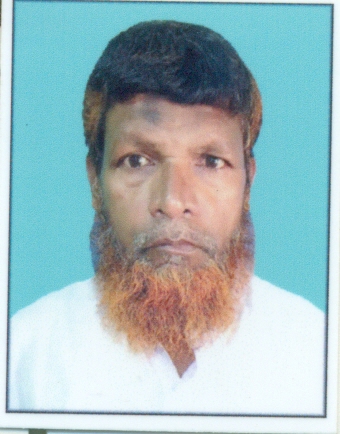
Hafizul Haque
Multi-Tasking Staff
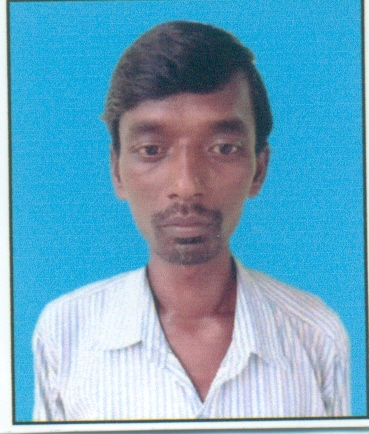
Suniram Marddi
Peon
Network Support Team
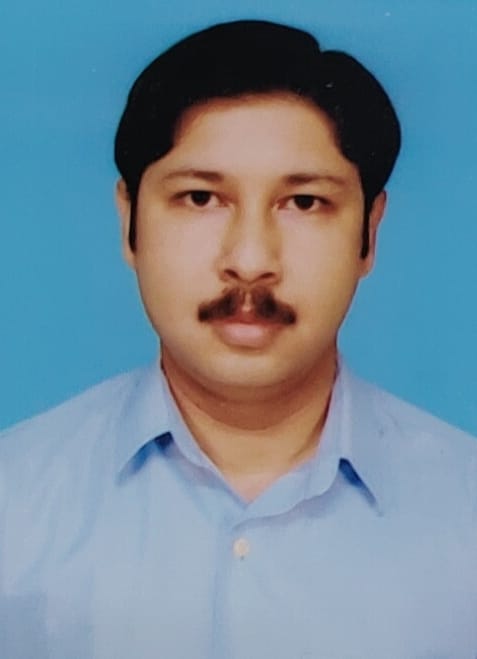
Rupam Das
Network Admin
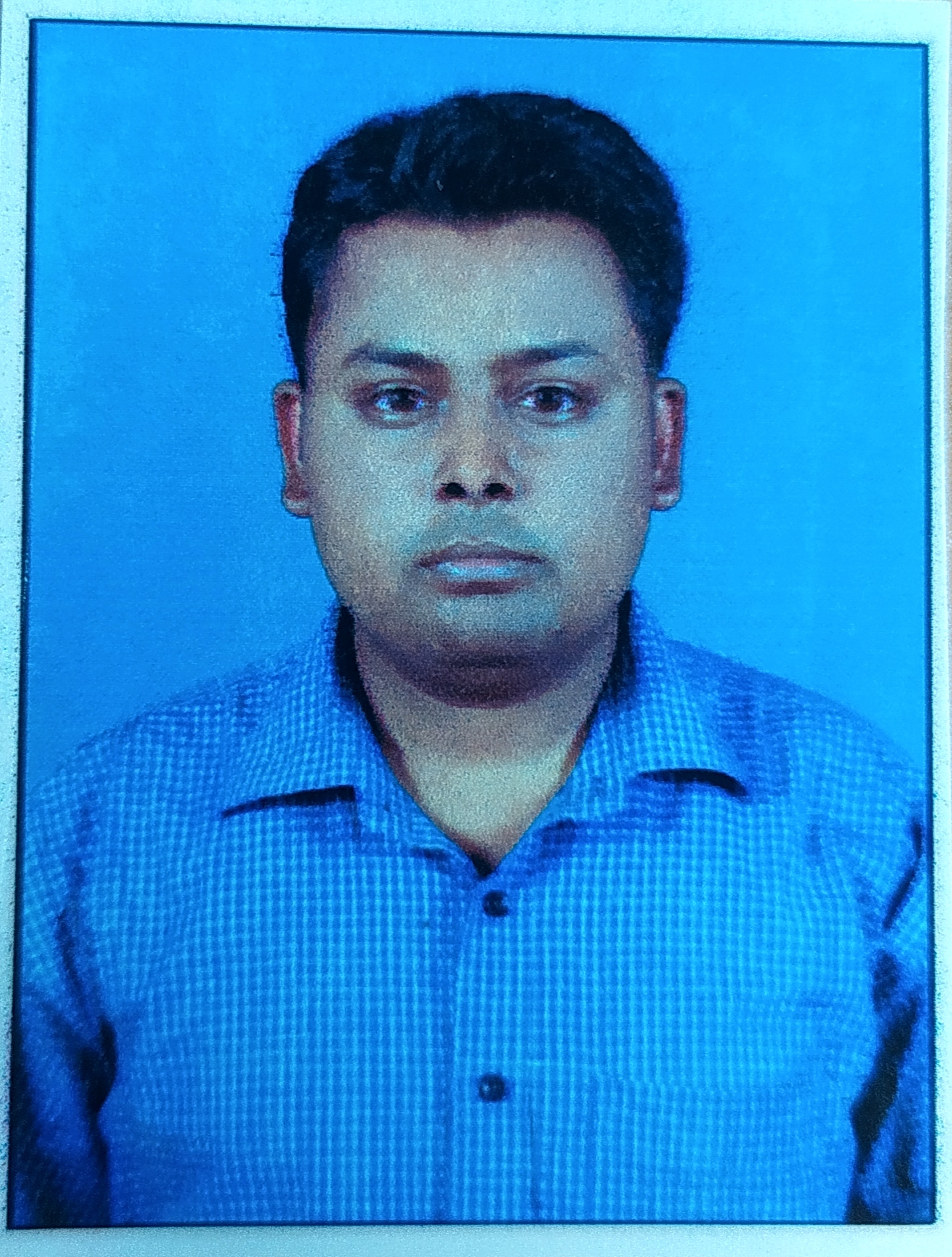
Pradip Adhikari
Network Admin
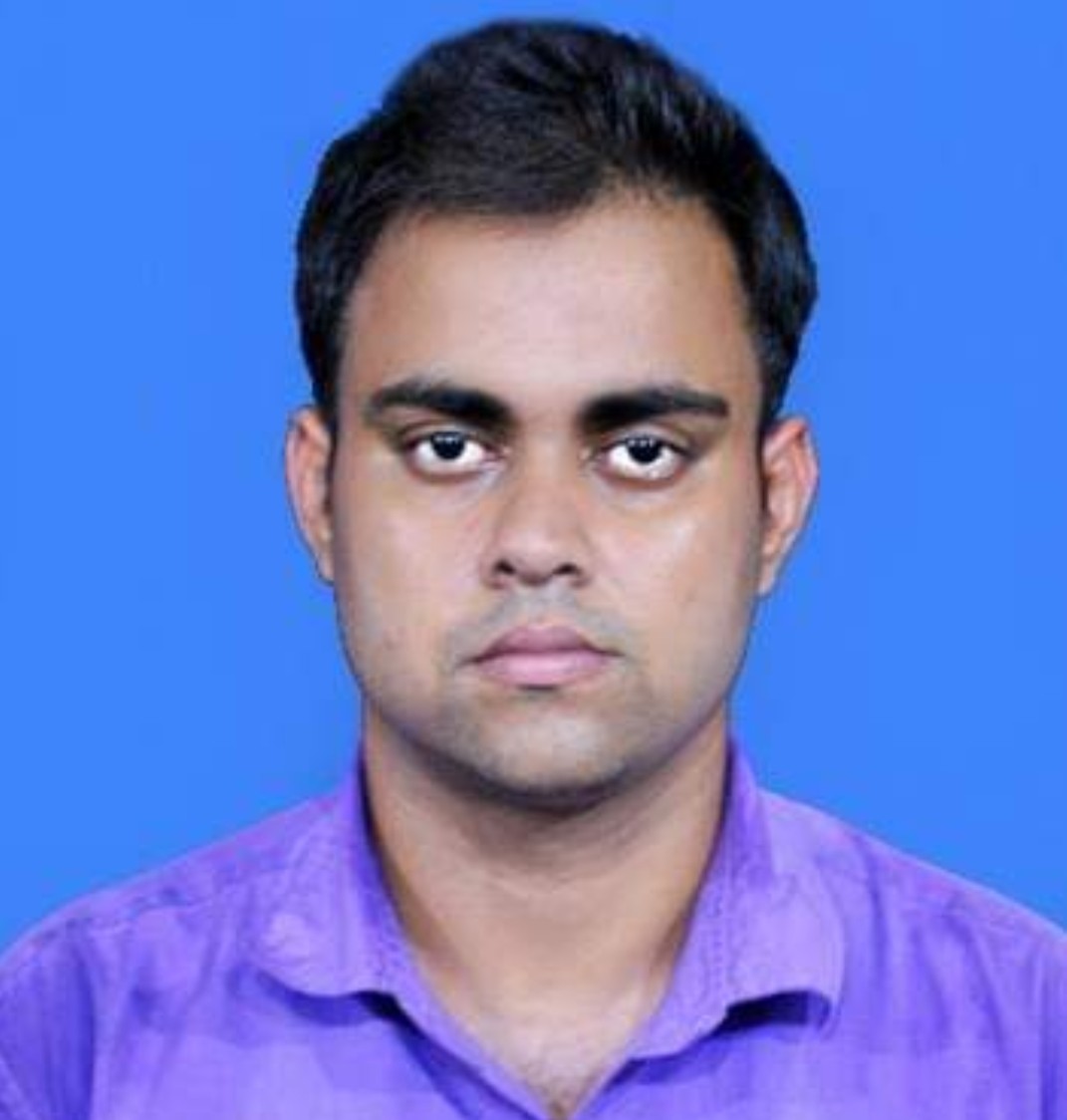
Subrata Mukherjee
Network Admin
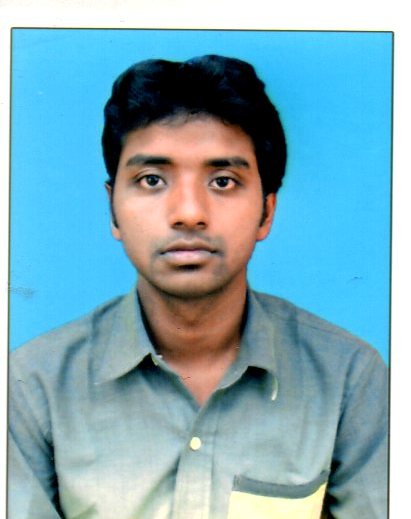
Supriyo Roy
Network Admin
Server Support Team
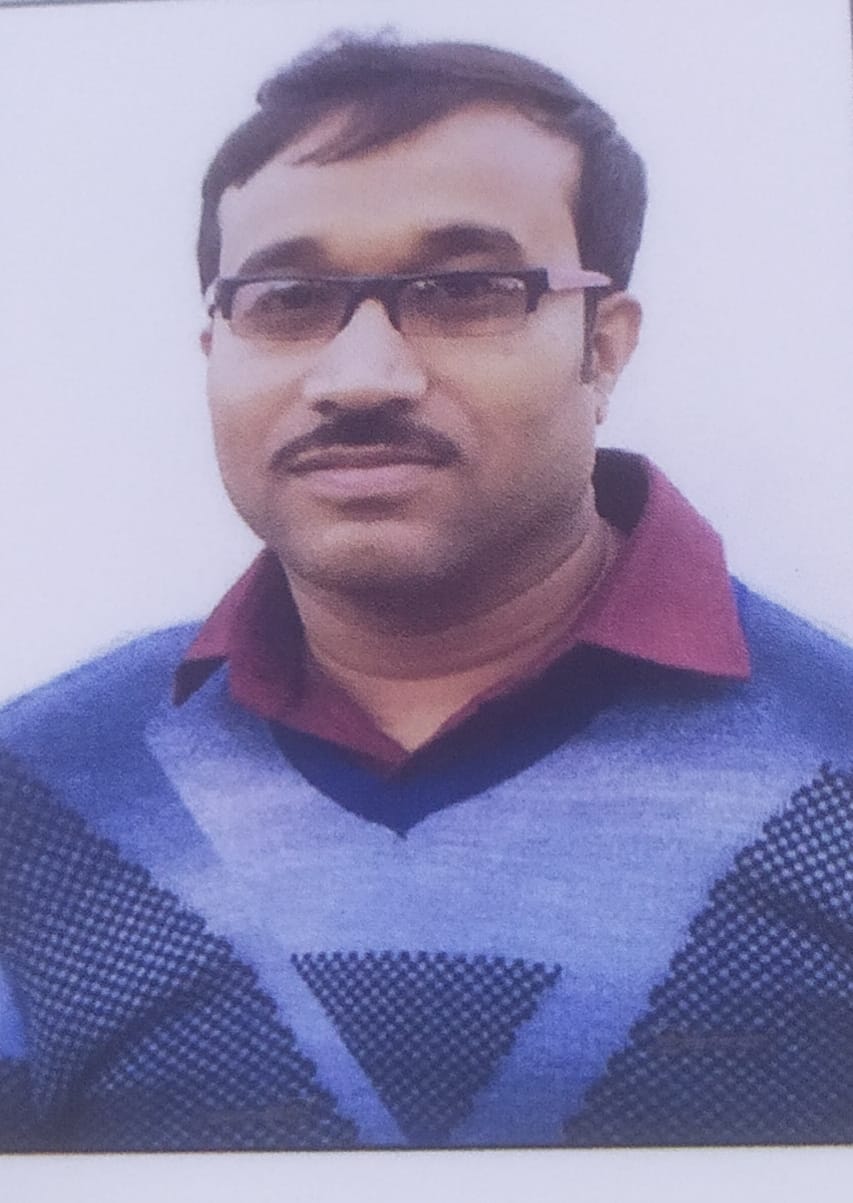
Asit Kumar Saha
System Admin
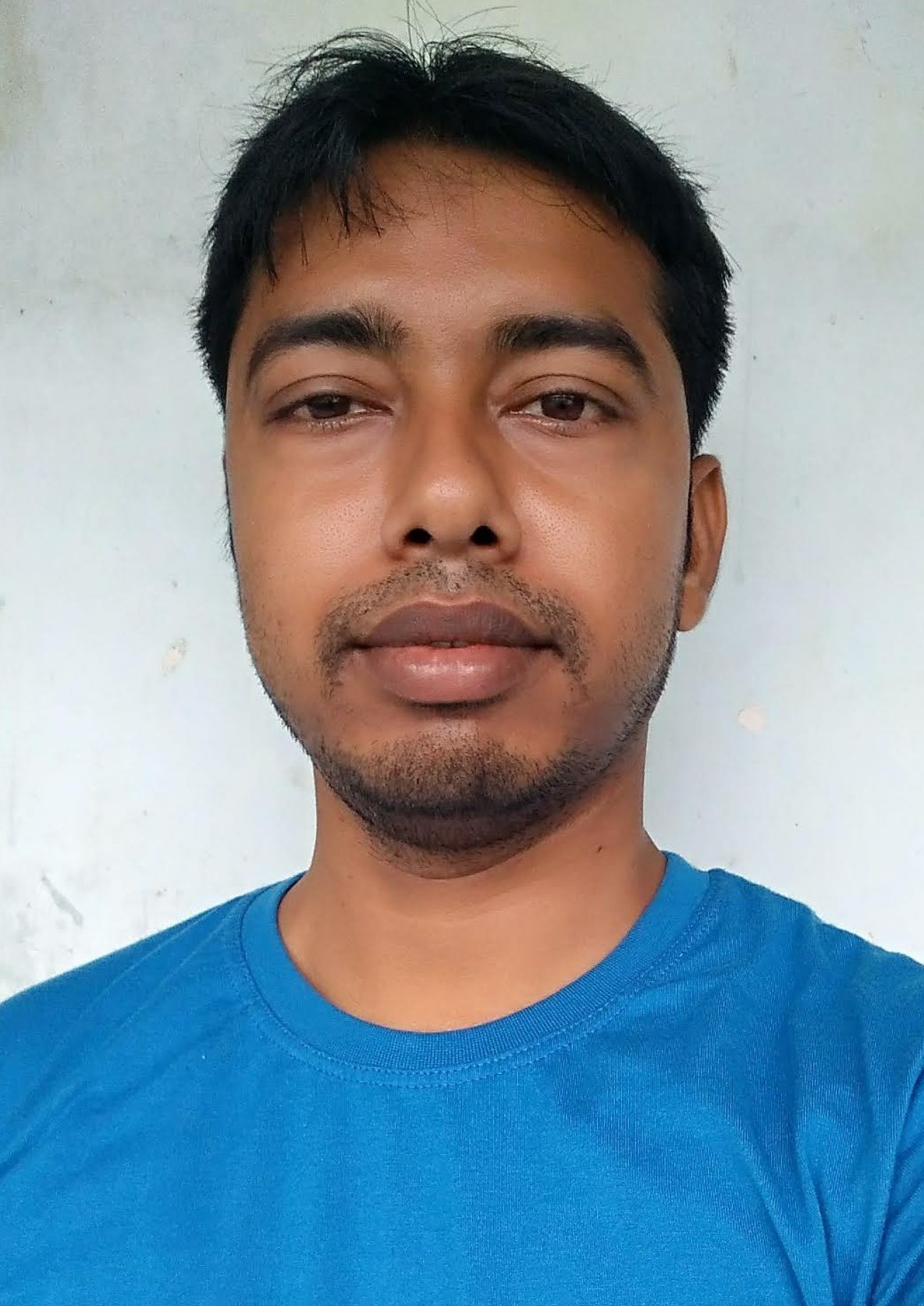
Hasibul Mondal
System Admin
HEAD
Dr. Bibhash Sen
Head
Computer Center
Email : hod.cc@nitdgp.ac.in